rhinoverse系列
|
|
在初始化项目中,相对重要基础的文件如下:
app/logic
文件夹:自定义分析函数;app/view
文件夹:自定义shiny模块;app/main.R
文件:shiny主体设计;app.R
文件:shiny的执行文件(不用修改);dependencies.R
文件:记录所需的依赖包,以更新lock.file;.Rprofile
文件:设置options
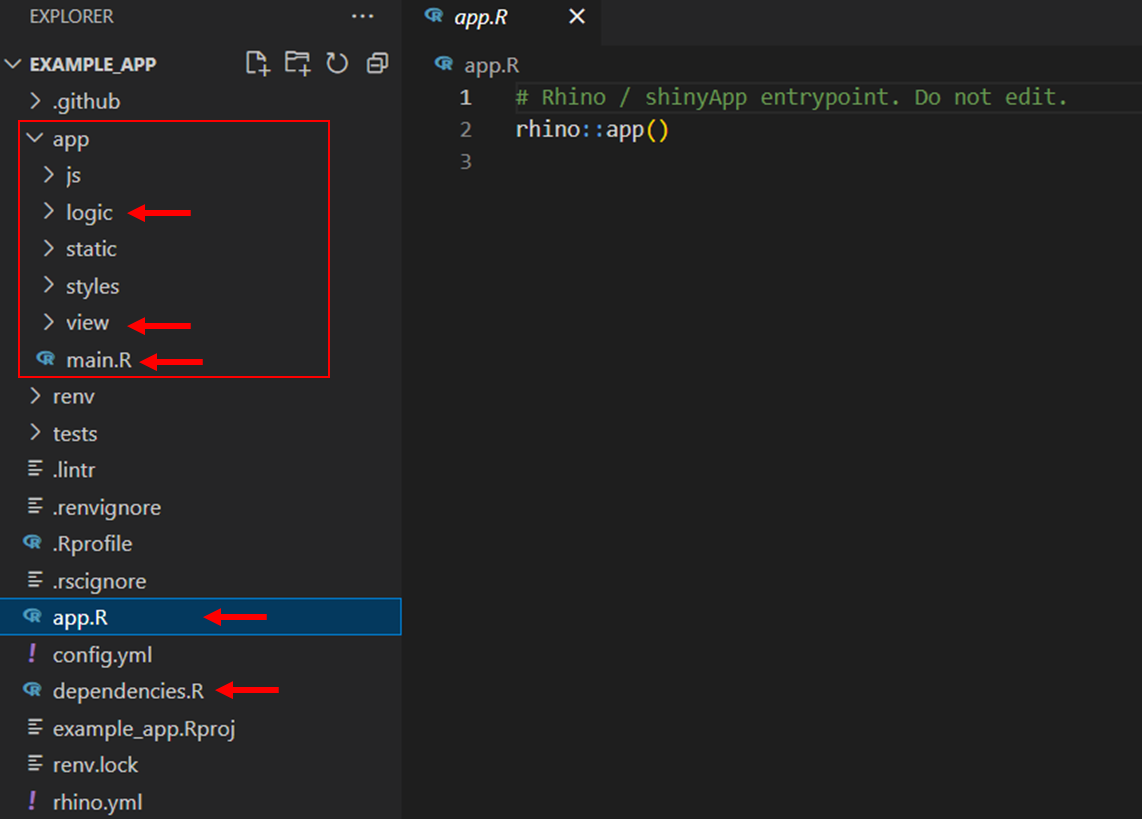
app/static
可以放置图片文件(img(src = "static/images/appsilon-logo.png")
), CSS、JS等修饰文件(对于JS,需要使用rhino::build_sass()
函数处理)
app/style
可以放置sass/scss等css预处理文件,需要使用build_sass()
函数处理
1. 安装包
pkg_install
安装相关依赖包;- 基于
renv::install()
函数在当前env环境内安装R包,并自动更新dependencies.R以及相应的lock.file;
|
|
2. 定义函数
- 在
app/logic
文件夹内定义R函数; - 一个文件内可以包含相关的多个函数,如下为
app/logic/func_iris.R
- 首先需要通过
box::use
函数引入所需要的工具包或特定函数,相关用法可以参见https://klmr.me/box/介绍。(可以类比Python中import pkg
orfrom pkg import aa, bb
更好地理解) - 然后在定义每个函数时,需要先加
#' @export
,再编写函数内容。其中使用导入的工具包里的某个函数或数据时需要使用$
,例如下面的datasets$iris
。 - 如下,共编写了两个iris相关的函数,
iris_data
返回表格;iris_plot
返回图形。
|
|
- 测试函数
|
|
3. 定义模块
- 在
app/view
文件夹内定义shiny模块,一个文件内包含一个模块(ui+server)。 - 首先需要使用
box::use()
加载所需工具包及特定函数,或自定义的函数。 - 然后按定义shiny模块的ui与server函数,同样需要添加
#' @export
语句。 - 如下,分别定义了两个模块,用于呈现表格与图形
app/view/mod_table.R
|
|
app/view/mod_plot.R
|
|
4. 编写main.R
- 接下来,根据已有的模块、函数等,设计总体的shiny页面的布局;
- 如下,为简单的
app/main.R
文件。与编写模块类似,首先加载需要的工具包与函数,然后编写ui与server内容。
|
|
5. 测试网页
- 调用 app.R文件,生成并测试网页;
- 然后,根据需要重复步骤1~4,修改或丰富app内容。
|
|